Last modified: Fri, 23 Apr 2010 12:08:30 +0300
Complete: ###################- (95%)
2.1 Introduction
2.2 Data Types
2.3 Identifiers
2.4 Variables
2.5 Constants
2.6 Basic Operators
2.7 Basic Strings
2.8 Header Files
2.9 Basic Intrinsic Functions
2.10 Exercises
2.1 Introduction
Almost all high level programming languages contain the concept of the data type, constants and variables.
In this section, we will learn how these fundamental elements are defined in C++. In addition,
an important part of the C++ the 'Header Files' and basic intinsic (library) functions will be considered.
An understanding of these basic concepts is essential for C++ and so the student is advised to study this section
of the tutorial before moving on to any other sections.
2.2 Data Types
A key component of a program is the use of objects that store data.
A data type determines the type of the data that will be stored, usually, in the computer memory (RAM).
C++ provides six fundamental data types:
char
int
float
double
bool
wchar_t
However, there are some qualifiers that can be put in front of the numerical data types
to form their derivatives:
short
long
signed
unsigned
Table 2.1 shows the fundamental data types in C++, as well as the range of values.
Table 2.1: Fundamental data types and their size and ranges in the memory. The numbers are evaluated for a 32-bit system.
Data Type |
Description |
Size (byte) |
Lower Limit |
Upper Limit |
char |
Character or small integer |
1 |
-128 |
127 |
unsigned char |
0 |
255 |
short int |
Short integer
| 2 |
-32,768 |
32,767 |
unsigned short int |
0 |
65,535 |
int |
integer |
4 |
-2,147,483,648 |
2,147,483,647 |
unsigned int |
0 |
4,294,967,295 |
long int |
Long integer |
8 |
-9,223,372,036,854,775,808 |
9,223,372,036,854,775,807 |
unsigned long int |
0 |
18,446,744,073,709,551,615 |
float |
Single precision floating point number (7 digits) |
4 |
-3.4e +/- 38 |
+3.4e +/- 38 |
double |
Double precision floating point number (15 digits) |
8 |
-1.7e +/- 308 |
+1.7e +/- 308 |
long double |
Quad precision floating point number (34 digits) [*] |
16 |
-1.0e +/- 4931 |
+1.0e +/- 4931 |
[*] only on 64 bit platforms. See also here.
You dont need to write int after using short and long keywords. Namely,
short s; // means "short int s";
long k; // means "long int k";
In addition, all integer types (int, long, short) come in two varieties: signed and unsigned. The idea here is that sometimes
you need negative numbers, and sometimes you don't. Integers without the word "unsigned" are assumed to be signed.
signed integers are either negative or positive. unsigned integers are always positive and so have a larger positive range.
2.3 Identifiers
An identifier is a string of alphanumeric characters. It is used for naming variables, constants, functions, stuctures and classes.
A valid identifier must begin with a letter or underscore (_), and can consist only of letters, digits, and underscores.
In addition, an identifier should not match with any C++ reserved keywords which are:
asm, auto, bool, break, case, catch, char, class, const, const_cast, continue, default, delete,
do, double, dynamic_cast, else, enum, explicit, export, extern, false, float, for, friend, goto, if,
inline, int, long, mutable, namespace, new, operator, private, protected, public, register, reinterpret_cast,
return, short, signed, sizeof, static, static_cast, struct, switch, template, this, throw, true, try, typedef,
typeid, typename, union, unsigned, using, virtual, void, volatile, wchar_t, while
According to these rules, the following are valid identifiers
mass, Force, pos12, speed_of_light, SpeedOfLight, isPrime
while the following are not
2ndBit, speed of light, yağmur, c++, float
Note that
C++ is case sensitive. That is, it distinguishes uppercase letters from lowercase letters.
So, Result and result are different identifiers.
|
2.4 Variables
A variable is a symbolic name indicating a location in your computer's memory where you can store a value which can later be retrieved.
A variable has to be a valid identifier. When you define a variable in C++, you must tell the compiler what kind of variable it is:
an integer, a character, and so forth. The general form of the declerations is:
where data-type is usually one of the fundamental data types and list is list of variables sperated by commas.
For example
int mass i, j;
double x, speed, dragForce;
when a variable is declared, you can initialize it in two alternative but equivalent ways
or
All variables must be declared before use. They can be declared either at start of program (like in C, Fortran) or
just before first use (like in java). Both usages are illustrated in Program 2.1.
02prg01.cpp: Decleration of variables
1:
2:
3:
4:
5:
6:
7:
8:
9:
10:
11:
12:
13:
14:
15:
16:
|
// Decleration of variables
#include <iostream>
using namespace std;
int main ()
{
short x = 22, y = 11, z;
z = x - y;
cout << "z = " << z << endl;
int p = 3;
int q = x*y*z - 2*p;
cout << "q = " << q << endl;
return 0;
} |
z = 11
q = 2656
|
In C++, a variable can have either local or global scope.
The scope (visibility) of local variables is limited to the block enclosed in braces ({}) where they are declared.
Global variables are declared outside of all blocks and their scope are the entire program, i.e. the main body of the source code.
The location of local and global variables are illustrated in Fig. 2.1.
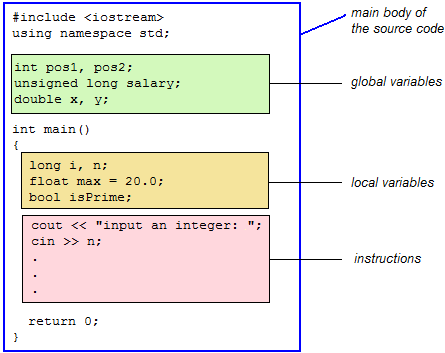
Fig 2.1: The location of example global and local variables in C++.
A program may have several variables with the same name as long as their scopes are nested or disjoint.
This is illustrated in Program 2.2.
02prg02.cpp: Nested and parallel scopes
1:
2:
3:
4:
5:
6:
7:
8:
9:
10:
11:
12:
13:
14:
15:
16:
17:
18:
19:
20:
|
// Nested and parallel scopes
#include <iostream>
using namespace std;
int k = 11; // this k is global
int main ()
{
int k = 22; // this k is local in main()
{
int k = 33; // this k is local in this block
cout << "Inside internal block: k = " << k << endl;
}
cout << "Inside main(): k = " << k << endl;
cout << "Global k = " << ::k << endl;
return 0;
} // end main() block |
Inside internal block: k = 33
Inside main(): k = 22
Global k = 11
|
There are three different variables named k in this program. The k at line 5 is a global,
so its scope extends through the file. The k at line 9 has scope limited to the main() block.
The last k in the block, nested within the main() block, hides the first and second k.
To access the global k in any block the scope resolution operator (::) is used as in line 17.
2.5 Constants
Sometimes you want to use a value that does not change, such as the number pi.
This is done by using const keyword as follows:
const float PI = 3.1415926, TWOPI = 2.0*PI;
const int EOF = -1;
You can define symbolic constants, that are not memory-consuming, by using the #define preprocessor directive.
For example:
#define PI 3.1415926
#define MAX 100
#define NEWLINE '\n'
Note that, by convension variable names use lowercase letters while constants use uppercase only.
Sometimes we want to assign numerical values to words, e.g. January = 1, February = 2, and so on.
C++ allows to define 'enumeration' constants with keyword enum.
For example
enum { RED, GREEN, BLUE };
is shorthand for
const int RED = 0, GREEN = 1, BLUE = 2;
Enumeration starts by default with zero but we can override this behaviour as follows:
enum { RED = 1, GREEN = 3, BLUE = 7 };
If not assigned explicitly, each value is one greater than previous. For example,
enum { RED = 1, GREEN, BLUE };
is equivalent to
enum { RED = 1, GREEN = 2, BLUE = 3 };
Numerical constants in C++ are called numerical literals. Each data type has its own literal.
Consider the following assignments:
i = 25; // 25 is an integer literal constant
r = 17.2; // 17.2 is a double literal constant
Actualy, literal constants can be divided in Integer Numerals, Floating-Point Numerals, Characters, Strings and Boolean Values.
By default, integer literals are of type int, and real literals are are of type double.
However, this can be changed by adding the suffix U (or u), L (or l) and F (or f) to the end of a literal.
So the following do not have the same meaning:
i = 25; // default int literal
i = 25U; // unsigned int literal
i = 25L; // long int literal
i = 25UL; // unsigned long literal
r = 17.2; // default double literal
r = 17.2L; // long double literal
r = 17.2F; // float literal
Integer literal constants can be represented by three different bases: base-10 (decimal), base-8 (octal) and base-16 (hexadecimal).
This is done by adding the prefix 0 (zero) for octal literals, and 0x for hexadecimal literals.
In the hexadecimal notation either capital letters (A, B, C, D, E and F)
or their lower case (a, b, c ,d, e and f) can be used.
According to this, the following are equivalent.
i = 75; // defult base-10
i = 0113; // base-8
i = 0x4B; // base-16
i = 0x4b; // base-16
Floating point literals express numbers with decimals and/or exponents. The symbol E or e is used in the exponent.
For example, the following notations are equivalent and can be used to represent the number 123.456.
x = 123.456; // decimal
x = 123.456e+0; // exponent
x = 1.23456E+2; // exponent
x = 1234.56e-1; // exponent
Here 1.23456e+2 is equivalent to 1.23456 x 10+2 in mathematical notation.
For string literals, we can use single quote for a character, and double quotes for a
one or more than one characters.
'A' // a single character
"B" // a single character
"Hello World" // a set of characters
There are aditional character literal called escape codes or escape sequences
which are preceded by a backslash (\),
like newline (\n) or tab (\t). Here you have a list of them:
Escape Code |
Description |
Example |
\a |
alert (beep) |
cout << "Error ! \a"; |
\b |
backspace |
cout << "No \bSpace"; |
\f |
form feed (page feed) |
cout << "Refresh...\f"; |
\n |
newline |
cout << "University of\nGaziantep"; |
\r |
carriage return |
cout << "University of\rGaziantep"; |
\t |
horizontal tab |
cout << x << '\t' << y; |
\v |
vertical tab |
cout << "Hello\vWorld"; |
\" |
double quote (") |
cout << "\"Hello\" said old man."; |
\' |
single quote (') |
cout << "This is Faraday\'s Law"; |
\\ |
single backslash (\) |
path = "C:\\Dev-Cpp\\Include"; |
\? |
question mark (?) |
cout << "How old are you\?" |
In C++, there are only two valid Boolean literals true and false.
These are expressed as values of type bool.
2.6 Basic Operators
Operators are special symbols that perform operations on the variables and constants.
Basic operators can be classified as follows:
Arithmetic Operators
These perform five fundamental operations
Operator |
Description |
Example |
Result |
+ |
Addition |
13 + 5 |
18 |
- |
Subtraction |
13 - 5 |
8 |
* |
Multiplication |
13 * 5 |
65 |
/ |
Division |
13 / 5 |
2 |
% |
Modulus (reminder from x/y) |
13 % 5 |
3 |
Note that, arithmetic expressions are evaluated with some rule called operator precedence.
Accordingly, all multiplications and divisions are evaluated first and additions and
subtractions are performed last. The following examples illustrates this order:
2 - 3 * 4 + 2 = -8
2 * 3 + 4 - 2 = 8
2 * (3 + 4) - 2 = 12
3 * 5 / 3 = 5
10 / 2 * 3 = 15
(5 + (11-5) * 2) * 4 + 9 = 77
Assignment Operator
Variables are given a value through the use of assignment operator (=) whose general form is.
For example
int x, y;
x = 2;
y = 5*x; // y = 10
x = x + 4; // x = 6
y = y/2; // y = 5
The value of an assignment can be used in another assignment.
m = (n = 66) + 9; // n = 66 and m = 75
x = y = 22; // x = 22 and y = 22
This is a chained assignment.
Compound Assignment Operators (+=, -=, *=, /=, %=)
These allow us to use assignment and an arithmetic operator together. The general form is:
variable operator= expression;
Examples are given below.
Operator |
Description |
Example |
Equivalent to |
+= |
add and assign |
x += 3 |
x = x + 3 |
-= |
subtract and assign |
x -= 5 |
x = x - 5 |
*= |
multiply and assign |
x *= 4 |
x = x * 4 |
/= |
divide and assign |
x /= 2 |
x = x / 2 |
%= |
find reminder and and assign |
x %= 9 |
x = x % 9 |
Increase and decrease by 1 (++, --)
The increase operator (++) and the decrease operator (--) increase or reduce by one the value stored in a variable.
The following are equivalent in functionality.
Note that
The ++ operator is used in the name "C++" because it increments the C programming language. That means, C++ has everyting that C has and more!
|
A characteristic of this operator is that it can be used both as a prefix and as a suffix.
Namely, a++ or ++a, but becareful the results may differ as illustrated below:
a = 5; // a = 5
b = a++; // b = a = 5, then a = 5+1 = 6
c = ++a; // a = 6+1 = 7, then c = a = 7
b = a++ means first assign a to b, and then increase a by 1.
Whereas c = ++a means first increase a by 1, then assign a to c.
Type Casting, Promotion and Demotion
Type casting refers to changing an entity of one data type into another.
This is done automatically by the compiler. For example,
int i, j;
double p, q;
i = 4/2; // i = 2
j = 5/2; // j = 2
p = 5/2; // p = 2.0
p = 5/2.0; // p = 2.5
q = i + p; // q = 2.0 + 2.5 = 4.5; (the compiler first converts 2 to a double 2.0, then adds 2.5)
Type casting operators allow you to convert a data of a given type to another. For example,
int i;
float f;
double d;
i = int(7.25); // i = 7
d = double(5); // d = 5.0
f = float(5)/2; // f = 2.5f
Promotion occurs whenever a variable or expression of a smaller type is converted to a larger type.
float a = 5; // 5 is an int constant, gets promoted to float
long b = 9; // 9 is an int constant, gets promoted to long int
double c = a; // a is a float, gets promoted to double
Demotion occurs whenever a variable or expression of a larger type gets converted to a smaller type.
int a = 7.5; // double gets down-converted to int; a = 7
double b = 1.3; //
float c = b; // double gets down-converted to float; c = 1.3f;
Note that demoting a double to a float generally involves a loss of precision, and promoting a float to a double
does not increase the precision of the value.
The sizeof() operator
The unary operator sizeof() is used to calculate the size in bytes of data types, variables, arrays or any object.
For example, the following code
int i;
double d;
cout << "sizeof(int) = " << sizeof(int) << " bytes" << endl;
cout << "sizeof(float) = " << sizeof(float) << " bytes" << endl;
cout << "sizeof(double) = " << sizeof(double) << " bytes" << endl;
cout << "sizeof(i) = " << sizeof(i) << " bytes" << endl;
cout << "sizeof(d) = " << sizeof(d) << " bytes" << endl;
will output
sizeof(int) = 4 bytes
sizeof(float) = 4 bytes
sizeof(double) = 8 bytes
sizeof(i) = 4 bytes
sizeof(d) = 8 bytes
The values in Table 2.1 are obtained by this operator.
More information can be found here.
2.7 Basic Strings
A string is a series of characters, such as "Hello World!".
C++ compilers include a class library that contains a large set of classes for data manipulation.
A standard component of a class library is a string class.
Although the string class is actually not a data type, it behaves like a fundamental type.
However, there are two differences: first, we need to include an additional header file <string>
in our source code, and second we need to use std namespace (as we did before).
A basic string application is demonstrated in Program 2.3.
02prg03.cpp: Using strings
1:
2:
3:
4:
5:
6:
7:
8:
9:
10:
11:
12:
13:
14:
15:
|
// Using strings
#include <iostream>
#include <string>
using namespace std;
int main ()
{
string s1, s2;
s1 = "This is a string";
s2 = "This is another string";
cout << s1 << endl << s2 << endl;
return 0;
} |
This is a string
This is another string
|
Note that, if you omit line 4 in this program, you have to use prefix std:: before string.
Strings can be initilized in a similar way as other variables.
string s = "This is a string";
or
string s("This is a string");
Strings can do all other basic operations that fundamental data types can. Examples are given below.
string s1, s2, s2, s4;
s1 = "centi";
s2 = "meter";
s3 = s1; // s3 = "centi";
s4 = s1 + s2; // s4 = "centimeter";
Note that, in C++, you can use C strings which are the array of chars
ending with a null character, '\0'.
char *s1 = "terabyte"; // sizeof(s1) is 8
char s2[] = "rpm"; // sizeof(s2) is 3
2.8 Header Files
The #include directive allows the program to use source code from another file. For example,
we have been using the following directive in our programs:
This refers to an external file named iostream, and tells the preprocessor to take the iostream file and insert in
the current program. The files that are included in other programs are called header files.
The C and C++ standard library traditionally declare their standard functions and constants in header files.
(see also: Wikipedia: C++ Standard Library).
Table 2.2 lists the C++ standard library headers.
Table 2.1: C++ standard library header files
C++ Standard Library |
Standard Template Library |
C Standard Library |
ios
iostream
iomanip
fstream
sstream |
vector
deque
list
map
set
stack
queue
bitset
algorithm
functional
iterator |
cassert
cctype
cerrno
climits
clocale
cmath
csetjmp
csignal
cstdarg
cstddef
cstdio
cstdint
cstdlib
cstring
ctime |
2.9 Basic Intrinsic Functions
An intrinsic or a library function is a function provided by the C++ language. Such functions are handled
specially by the compiler. Each library function is defined in an header file. Here, some important and useful functions are
given.
Some C++ library mathematical functions and constants defined in <cmath>
Function Decleration |
Description |
Example |
Result |
double fabs(double x); |
absolute value of real number, |x| |
fabs(-4.0) |
4.0 |
int floor(double x); |
round down to an integer |
floor(-2.7) |
-3 |
int ceil(double x); |
round up to an integer |
ceil(-2.7) |
-2 |
double sqrt(double x); |
square root of x |
sqrt(4.0) |
2.0 |
double pow(double x, double y); |
the value of xy |
pow(2., 3.) |
8.0 |
double exp(double x); |
the value of ex |
exp(2.0) |
7.38906 |
double log(double x); |
natural logarithm, logex = lnx |
log(4.0) |
1.386294 |
double log10(double x); |
base 10 logarithm, log10x = logx |
log10(4.0) |
0.602060 |
double sin(double x); |
sinus of x (x is in radian) |
sin(3.14) |
0.001593 |
double cos(double x); |
cosine of x (x is in radian) |
cos(3.14) |
-0.999999 |
double tan(double x); |
tangent of x (x is in radian) |
tan(3.14) |
-0.001593 |
double asin(double x); |
arc-sine of x in the range [-pi/2, pi/2] |
asin(0.5) |
0.523599 |
double acos(double x); |
arc-cosine of x in the range [-pi/2, pi/2] |
acos(0.5) |
1.047198 |
double atan(double x); |
arc-tangent of x in the range [-pi/2, pi/2] |
atan(0.5) |
0.463648 |
M_PI |
constant pi |
myPI = M_PI |
3.141592... |
M_E |
constant e |
x = M_E |
2.718281... |
Some standard C++ library functions and constant defined in <cstdlib>
Function Decleration |
Description |
Example |
Result |
int abs(int x); |
absolute value of integer number, |x| |
abs(-4) |
4 |
int atoi(const char *s); |
converts string to integer |
atoi("-1234") |
-1234 |
double atof(const char *s); |
converts a string to double |
atof("123.54") |
123.54 |
void exit(int status); |
can be used to abort the program |
exit(1) |
program aborts |
int rand(void); |
Returns a random integer between 0 and RAND_MAX |
rand() |
1048513214 |
RAND_MAX |
The largest number rand() will return |
x = RAND_MAX |
2147483647 |
2.10 Exercises
- How many data types are in C++?
- What is the difference between short int and int?
- What is an identifier?
- What is the difference between an integer variable and a floating-point variable?
- What are the advantages of using a symbolic constant rather than a literal constant?
- What are the values of s and p after the compound assignment in the following code?
int k = 2, s = 3, p = 4;
s += k++;
p *= ++k;
- Given the following enum, what is the value of TE?
enum { EP = 1, FE, IE = 4, EE, ME, TE, CE = 9 };
- Which of the following variable names are invalid?
Age !ex R79J 3Vec totalIncome __Invalid üzümSayısı
|